Steem Developer Portal
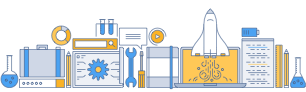
PY: Reblogging Post
We will show how to reblog or resteem post using Python, with username and posting private key.
Full, runnable src of Reblogging Post can be downloaded as part of the PY tutorials repository.
Tutorial will also explain and show you how to sign/broadcast transaction on Steem blockchain using the steem-python library.
Intro
Steem python library has built-in function to commit transaction and broadcast it to the network.
Steps
- App setup - Library install and import
- Post list - List of posts to select from trending filter
- Enter user credentials - Enter user credentails to sign transaction
1. App setup
In this tutorial we use 3 packages, pick
- helps us to select filter interactively. steem
- steem-python library, interaction with Blockchain. pprint
- print results in better format.
First we import all three library and initialize Steem class
import pprint
from pick import pick
# initialize Steem class
from steem import Steem
s = Steem()
2. Post list
Next we will fetch and make list of accounts and setup pick
properly.
query = {
"limit":5, #number of posts
"tag":"" #tag of posts
}
# post list from trending post list
posts = s.get_discussions_by_trending(query)
title = 'Please choose post to reblog: '
options = []
# post list
for post in posts:
options.append('@'+post["author"]+'/'+post["permlink"])
This will show us list of posts to select in terminal/command prompt. And after selection we will get formatted post as an option
variable.
3. Enter user credentials
Next in order to sign transaction, application asks for username and posting private key to sign transaction and broadcast it.
# get index and selected post
option, index = pick(options, title)
pprint.pprint("You selected: "+option)
account = input("Enter your username? ")
wif = input("Enter your Posting private key? ")
# commit or build transaction
c = Commit(steem=Steem(keys=[wif]))
# broadcast transaction
c.resteem(option, account=account)
That’s it, if transaction is successful you shouldn’t see any error messages, otherwise you will be notified.
To Run the tutorial
- review dev requirements
- clone this repo
cd tutorials/14_reblogging_post
pip install -r requirements.txt
python index.py
- After a few moments, you should see output in terminal/command prompt screen.